The Elastos Wiki
The official source of truth for the Elastos ecosystem, providing comprehensive documentation, community resources, and instructional guides for those interested in learning about or building on Elastos.
Browse the Wiki by Topic
Understanding Elastos
What is Elastos?
Learn the basics about Elastos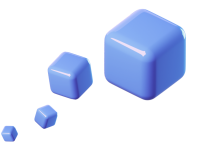
Multichain Architecture
Learn about our blockchain stack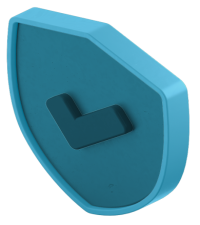
Sovereign Identities
Learn about decentralized identifiers (DIDs)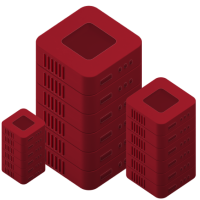
Data Vaults
Own your data with off-chain storage vault solutions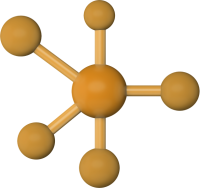
P2P Network
Safe network for transmitting data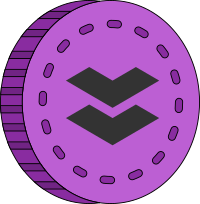
Token
Learn about the ELA token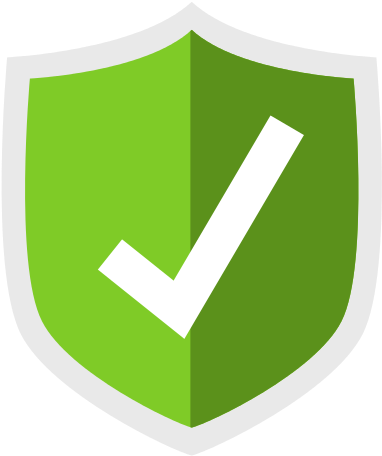
Consensus
Learn how the network is securedDeveloper Documentation
Quick Start
Creating your first dAppInteract with DIDs
Use DIDs for your dAppsBuild a Contract
Learn how to deploy contractsStore User Data
Learn how to access Hive data vaults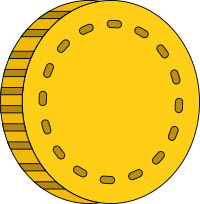
Fungible Tokens
Learn how to create tokens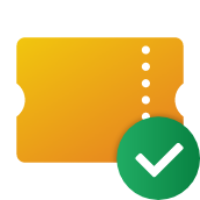
Non-Fungible Tokens
Enter the NFT spaceConnect a Web Frontend
Learn how to build a web dAppDeveloper Tools
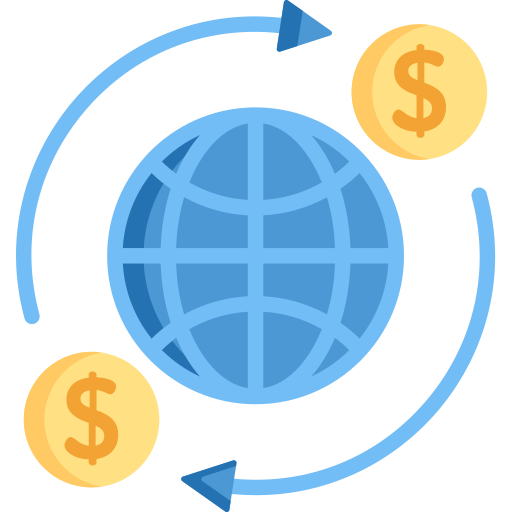
Public Endpoints
Interact with Elastos blockchainsConnectivity SDK
Interact with Elastos using TypescriptMainchain APIs
Use the Mainchain CLI or RPC APISmart Chain APIs
Use the ESC CLI or RPC API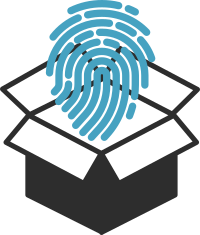
Identity SDKs
Work with DIDs using JS, C/C++, Java, or SwiftHive SDKs
Interact with Hive using JS, Java, or Swift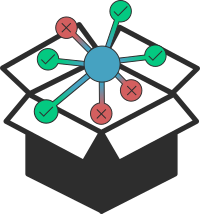
Carrier SDKs
Interact with CarrierOther Resources
Here are more sites from our ecosystem that can help you to learn more about Elastos.
Contact us
If you have any questions, or simply would want to chat with us, please do through one of our official channels.